Dear Miller,
Your script doesn’t specify any pixels as transparent. That’s why the output png will output flat colors. Setting up transparency is done in the fourth channel of the evalscript return statements, and the way to do it depends on which pixels you want transparent. You will also have to change the number of bands in output
of the function setup
to 4.
If for example you want all the pixels with NDVI lower than 0.3 transparent and other pixels colored as you chose, you could use 0 in the fourth channel to tell the script to not display these pixels, and 1 to tell the script to display the others. Like so:
//VERSION=3
function setup() {
return {
input: ["B05","B04"],
output: [
{ id:"default", bands: 4 }
]
};
}
function evaluatePixel(sample) {
let ndvi = (sample.B05 - sample.B04) / (sample.B05 + sample.B04)
if (ndvi < 0.3) return [0, 0, 0, 0]
else if (ndvi < 0.35) return [0.38, 0.59, 0.21, 1]
else if (ndvi < 0.4) return [0.31, 0.54, 0.18, 1]
else if (ndvi < 0.45) return [0.25, 0.49, 0.14, 1]
else if (ndvi < 0.5) return [0.19, 0.43, 0.11, 1]
else if (ndvi < 0.55) return [0.13, 0.38, 0.07, 1]
else if (ndvi < 0.6) return [0.06, 0.33, 0.04, 1]
else return [0, 0.27, 0, 1]
}
If you want all no-data pixels transparent, use dataMask. dataMask will recognize which pixels have no data, and if you put it into the fourth channel, these pixels will become transparent. dataMask is a band, so make sure to add it to input bands.
//VERSION=3
function setup() {
return {
input: ["B05","B04", "dataMask"],
output: [
{ id:"default", bands: 4 }
]
};
}
function evaluatePixel(sample) {
let ndvi = (sample.B05 - sample.B04) / (sample.B05 + sample.B04)
if (ndvi < -0.5) return [0.05, 0.05, 0.05, sample.dataMask]
else if (ndvi < -0.2) return [0.75, 0.75, 0.75, sample.dataMask]
else if (ndvi < -0.1) return [0.86, 0.86, 0.86, sample.dataMask]
else if (ndvi < 0) return [0.92, 0.92, 0.92, sample.dataMask]
else if (ndvi < 0.025) return [1, 0.98, 0.8, sample.dataMask]
else if (ndvi < 0.05) return [0.93, 0.91, 0.71, sample.dataMask]
else if (ndvi < 0.075) return [0.87, 0.85, 0.61, sample.dataMask]
else if (ndvi < 0.1) return [0.8, 0.78, 0.51, sample.dataMask]
else if (ndvi < 0.125) return [0.74, 0.72, 0.42, sample.dataMask]
else if (ndvi < 0.15) return [0.69, 0.76, 0.38, sample.dataMask]
else if (ndvi < 0.175) return [0.64, 0.8, 0.35, sample.dataMask]
else if (ndvi < 0.2) return [0.57, 0.75, 0.32, sample.dataMask]
else if (ndvi < 0.25) return [0.5, 0.7, 0.28, sample.dataMask]
else if (ndvi < 0.3) return [0.44, 0.64, 0.25, sample.dataMask]
else if (ndvi < 0.35) return [0.38, 0.59, 0.21, sample.dataMask]
else if (ndvi < 0.4) return [0.31, 0.54, 0.18, sample.dataMask]
else if (ndvi < 0.45) return [0.25, 0.49, 0.14, sample.dataMask]
else if (ndvi < 0.5) return [0.19, 0.43, 0.11, sample.dataMask]
else if (ndvi < 0.55) return [0.13, 0.38, 0.07, sample.dataMask]
else if (ndvi < 0.6) return [0.06, 0.33, 0.04, sample.dataMask]
else return [0, 0.27, 0, sample.dataMask]
}
See more about transparency in our transparency guide.
If this still doesn’t work, make sure you’re actually outputting a PNG output and not a JPEG. You can see this in the request body if you’re working with the API, or, if you’re downloading from EO Browser, check the image format in download.
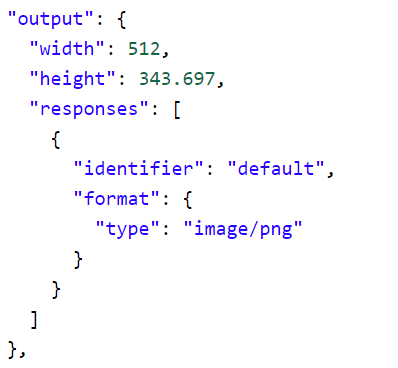
I hope this helps.
All the best,
Monja